Matlab 基礎知識-4
Matlab 的資料類型
Matlab 分為以下幾種資料型態
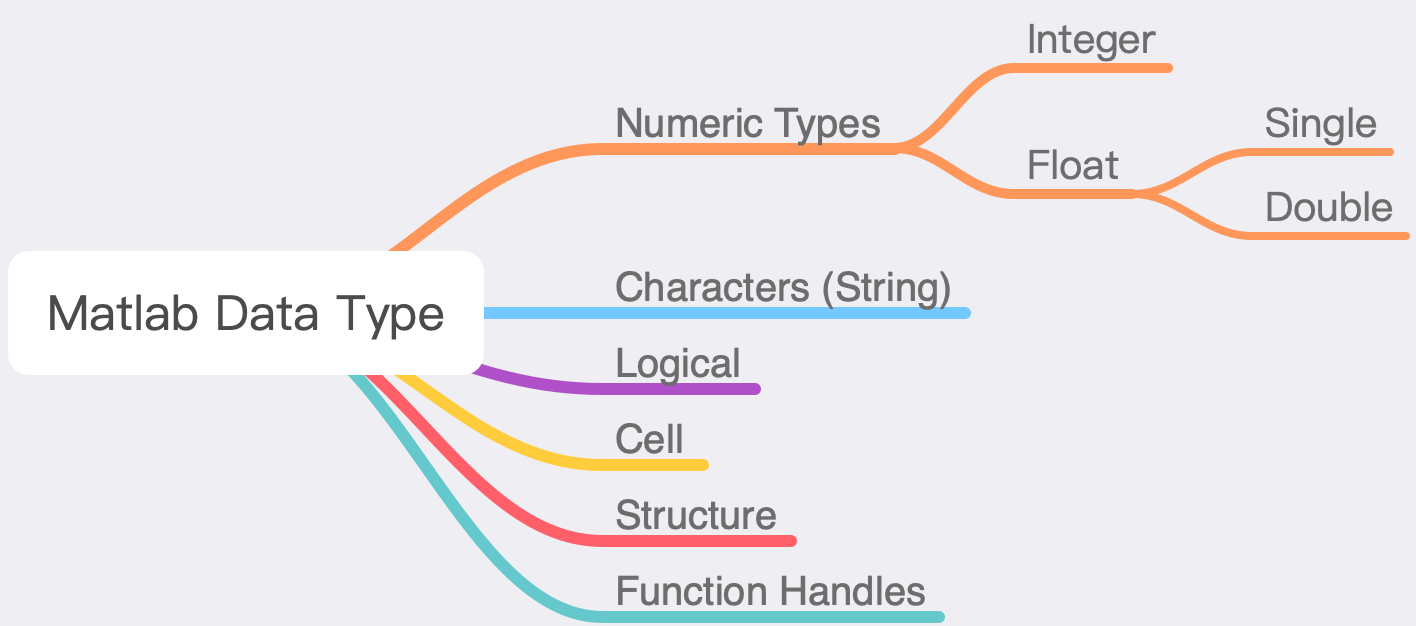
Numeric Type
數值類型 (Numeric Type) 又可依數值型態分為整數 (Integer) 及浮點數 (Float)。
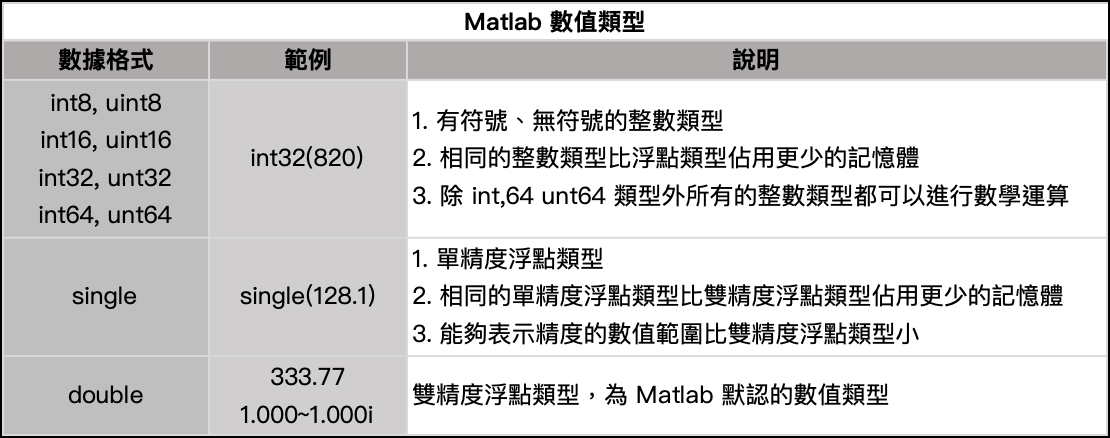
整數 (Integer) 下分有無符號及佔用位元組數分為 8 種整數類型。
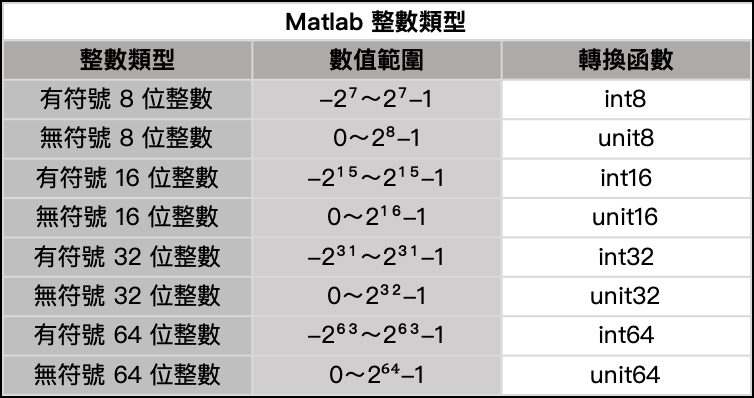
浮點數 (Float) 下分單精度 (Single)及雙精度 (Double),其中雙精度為 Matlab 默認存儲所有數值的型態。
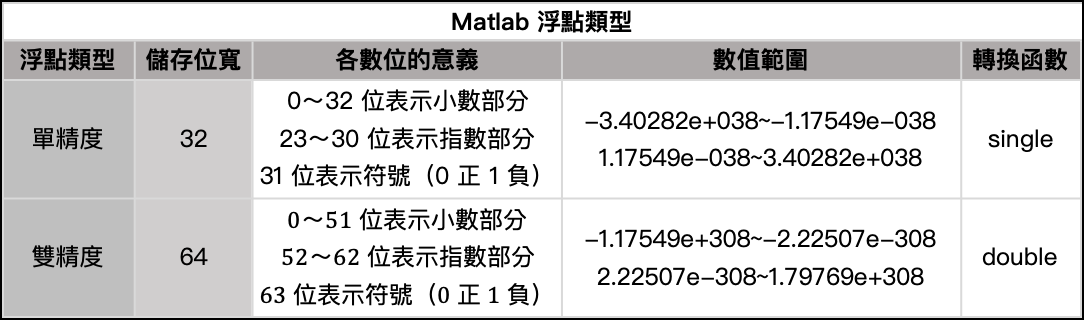
Numeric Type 會影響與記憶體的佔用大小,與 Double 相比,以 Integer 和 Single 形式儲存的數據會更節省記憶體空間。
Logical
指的是布林值 (Boolean) 的數據與數據之間的邏輯 (Logical) 關係,在 Matlab 中把任何非零數值當為真,把零當為假,把所有關係以邏輯表達式輸出:對於真 (True) 輸出為 1;對於假 (False) 輸出為 0。
在 Matlab 中邏輯運算所使用的運算符號如下表所示。
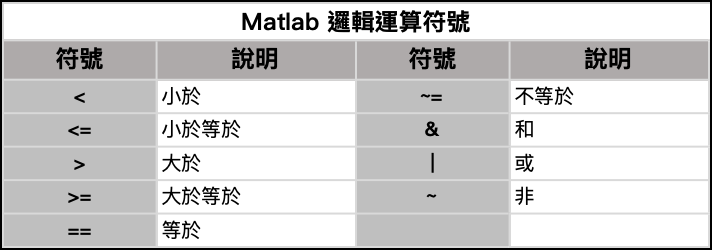
Characters (String)
字元 (Characters) 和字串 (String) 主要用於儲存本文數據;一個英文字母、數字或其他的符號 (包含空白符號),我們稱它為 Characters,而 String 就是一段文字,是由一個或多個 Characters 所組成的。
在 Matlab 中,一個 String 是被視為一個列向量 (Row Vector) 所儲存,而此一字串中的每一個 Characters,是以其 ASCII 碼的形式存放於此 Row Vector 中的元素 (Element),每個字元會佔用 2 Bytes 的記憶體空間。
在 Matlab 中是使用單引號 ’
做為 String 符號,如果在 String 中要顯示單引號,在字串中需使用雙單引號 e.g. ’shuwn''s program'
。
1 | >> string = 'hello world' |
另外,在 Matlab 中較為特殊的是,String 可視為行向量 (Column)
1 | >> b='hello' |
此外,String 也可視為 Matrices,但這種用法很罕見,同時要求各行 String 長度一樣,否則將違反矩陣行列數規定。
1 | >> a=['ab';'cd'] |
當然 String 的值也可以是特殊符號,比如 ’,’
就定義了逗號,而最特殊的就是定義單引號,因為單引號會和字元串定義中的單引號混淆,因此 Matlab 中用兩個單引號表示一個單引號,如下面範例所示。
1 | >> a='''' |
Cell
細胞型資料結構 (Cell) 其作用是可以儲存不同的資料型態於同一個陣列中,它是一種廣義矩陣,可以包含 Matlab 任意類型的數據,通常包含 String、Numeric 組合或不同大小的 Matrices,使用 { }
來創建、顯示。
1 | >> c = {'x', [1:3;4:6;7:9]; 10, pi} |
Structure
結構 (Structure) 是由單一或多個資料欄位 (Field) 所組成,每個 Field 可以容納 Matlab 任意類型的數據,以下是一組範例說明。
1 | %% 以下為 Structure 的建立,設置 Structure 名稱為 student,在 student 下為各個 Field 設置名稱、內容,其中內容由 Element 所組成 |
點選 Workspace 的 student value,我們在 Variables 視窗中可以看到 student 的 Structure 內容是各種類型的數據所組成。
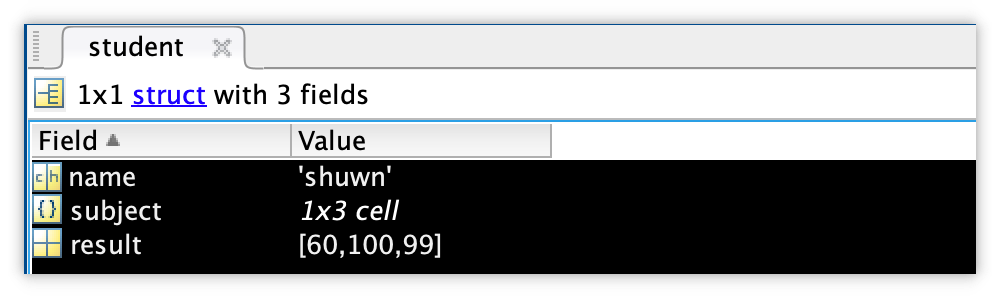
Function Handle
函式句柄 (Function Handle) 是一種能夠提供對函式 (Function) 間接呼叫的一種方式,它屬於 Matlab 一種數據類型,是一種 Variables,它可以保有所呼叫函式的所有要資訊,而且在呼叫函式時並不需要考慮所在呼叫位置,以下為 Function Handle 的基本架構。
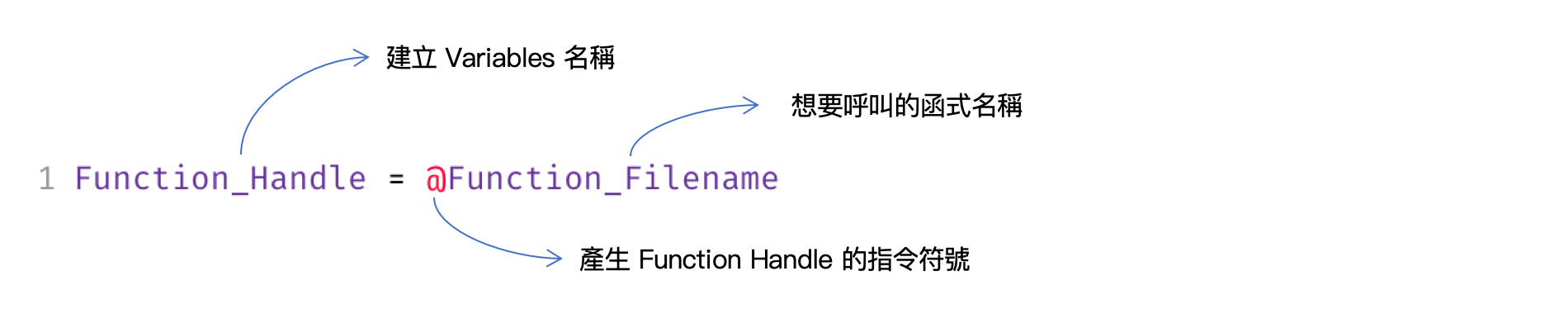
- Function Handle 是透過在函式名稱前添加一個 @ 符號來創建
- 其 Function Handle 所創建的 Variables,仍比照 Variables 的命名方式
以下為一個自定義的簡單 Functions File
1 | function y = my_function(x) |
我們在 Command Windows 中下達指令
1 | >> fh1 = @my_function %fh1 即為創建的 Functions File |
另一種無 Arguments 的 Function Handle 使用方式
1 | >> fh2 = @randn %使用 Matlab 內建 Functions 「randn」 創建 Functions File |
Function Handle 的使用重點
- 將函式的存取資訊傳遞給其他的函式 (可從main-Function 以外的地方,呼叫 local-Function)。
- 增進須重複運算的程式執行效率。
- 允許局部函式與專用函式更廣泛的存取範圍。
- 包含更多函式在同一 m-File 裡以方便 Functions 檔案的管理。